In this guide, we’ll explore how to use the CSS Flexbox layout to position content inside a container, whether it’s an element or plain text. We’ll go through examples for each case to illustrate the process. Importantly, the examples will concentrate on a single item within a Flexbox container, aiming to distinctly illustrate the diverse positioning options provided by Flexbox.
The Flexbox layout model provides the ability to align content in nine primary positions:
- Top Left;
- Top Center;
- Top Right;
- Center Left;
- True Center;
- Center Right;
- Bottom Left;
- Bottom Center;
- Bottom Right.
Flexbox’s extensive property range allows for more elaborate positioning beyond the basic nine described here. This article focuses on these common positions for ease of understanding. The next section delves deeper into Flex Container CSS, specifically on how to effectively style select options in CSS.
Styling a Container for Element Positioning with Flexbox
Begin with a basic HTML structure that includes a container and a content element:
<div class="content-container">
<span>Element with text content</span>
</div>
For clarity, let's implement some simple styling:
.content-container {
background: lightgreen;
}
.content-container span {
background: lightcoral;
}
To arrange content across nine distinct positions, the container must have dimensions that exceed the size of the content it encloses.
When the display property of a container is designated as flex, it naturally extends its width to 100%. The container’s height is determined by the height of the content within it, which can vary between items, or it can be manually defined for uniformity.
While the use of the flex-direction property is not strictly required, it does play a role in the ultimate placement of the content.
flex-direction is a CSS property that orchestrates the layout of flex items within their container, deciding the primary orientation axis—either horizontal or vertical—and their sequential direction.
CSS:
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
}
When utilizing flex-direction: row, horizontal placement within the container is controlled by the justify-content property, while vertical alignment is managed by align-items.
Conversely, with flex-direction: column, align-items dictates the horizontal orientation, and justify-content determines the vertical alignment.
The justify-content and align-items properties share the same set of values for positioning:
- flex-start aligns items to the start of the flex container, translating to left in horizontal orientation and top in vertical;
- center places items at the center, both horizontally and vertically;
- flex-end aligns items to the end of the flex container, translating to right in horizontal orientation and bottom in vertical.
Positioning at the Top Left
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: flex-start; /* horizontal position */
align-items: flex-start; /* vertical position */
}
Positioning at the Top Center
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: center; /* horizontal position */
align-items: flex-start; /* vertical position */
}
Positioning at the Top Right
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: flex-end; /* horizontal position */
align-items: flex-start; /* vertical position */
}
Positioning at the Center Left
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: flex-start; /* horizontal position */
align-items: center; /* vertical position */
}
Positioning at the Center Center
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: center; /* horizontal position */
align-items: center; /* vertical position */
}
Positioning at the Center Right
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: flex-end; /* horizontal position */
align-items: center; /* vertical position */
}
Positioning at the Bottom Left
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: flex-start; /* horizontal position */
align-items: flex-end; /* vertical position */
}
Positioning at the Bottom Center
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: center; /* horizontal position */
align-items: flex-end; /* vertical position */
}
Positioning at the Bottom Right
.content-container {
background: lightgreen;
height: 100px;
display: flex;
flex-direction: row;
justify-content: flex-end; /* horizontal position */
align-items: flex-end; /* vertical position */
}
Styling a Container with Textual Content
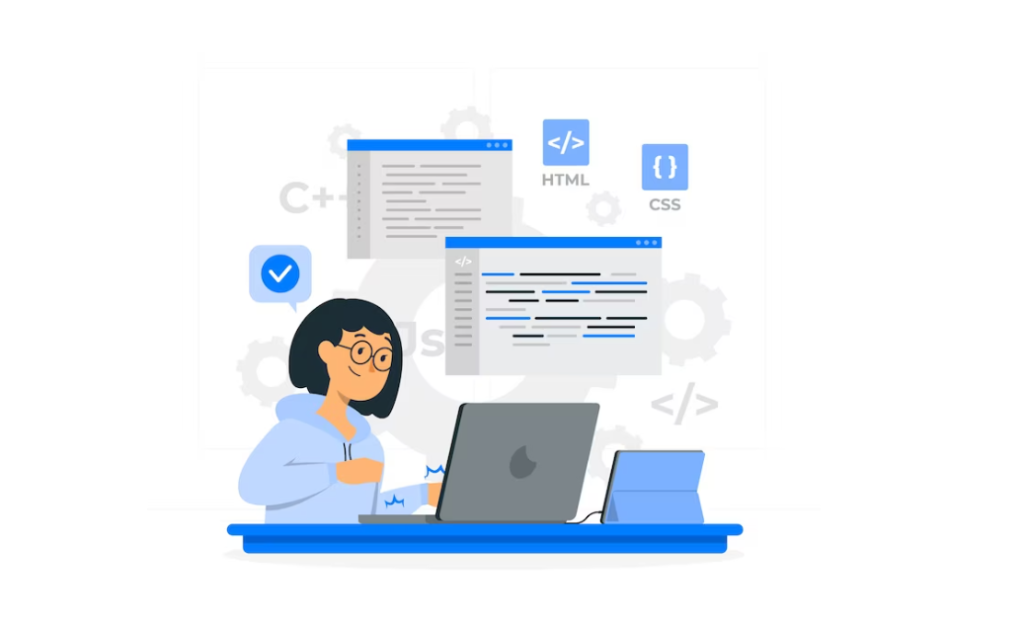
In this example, a container directly holds text.
<button class="button-container">Hello World</button>
To visually enhance this, apply some fundamental CSS.
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
cursor: pointer;
}
In this instance, the button element is utilized, hence setting the display property to inline-flex is appropriate. The flex-direction is assigned column to facilitate vertical positioning of the content.
Given that the default styling is removed from the button, causing its dimensions to match its content, adding width and height properties will provide additional space for the content.
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
}
Button content Top Left position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: flex-start; /* vertical position */
align-items: flex-start; /* horizontal position */
}
Button content Top Center position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: flex-start; /* vertical position */
align-items: center; /* horizontal position */
}
Button content Top Right position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: flex-start; /* vertical position */
align-items: flex-end; /* horizontal position */
}
Button content Center Left position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: center; /* vertical position */
align-items: flex-start; /* horizontal position */
}
Button content Center Center position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: center; /* vertical position */
align-items: center; /* horizontal position */
}
Button content Center Right position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: center; /* vertical position */
align-items: flex-end; /* horizontal position */
}
Button content Bottom Left position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: flex-end; /* vertical position */
align-items: flex-start; /* horizontal position */
}
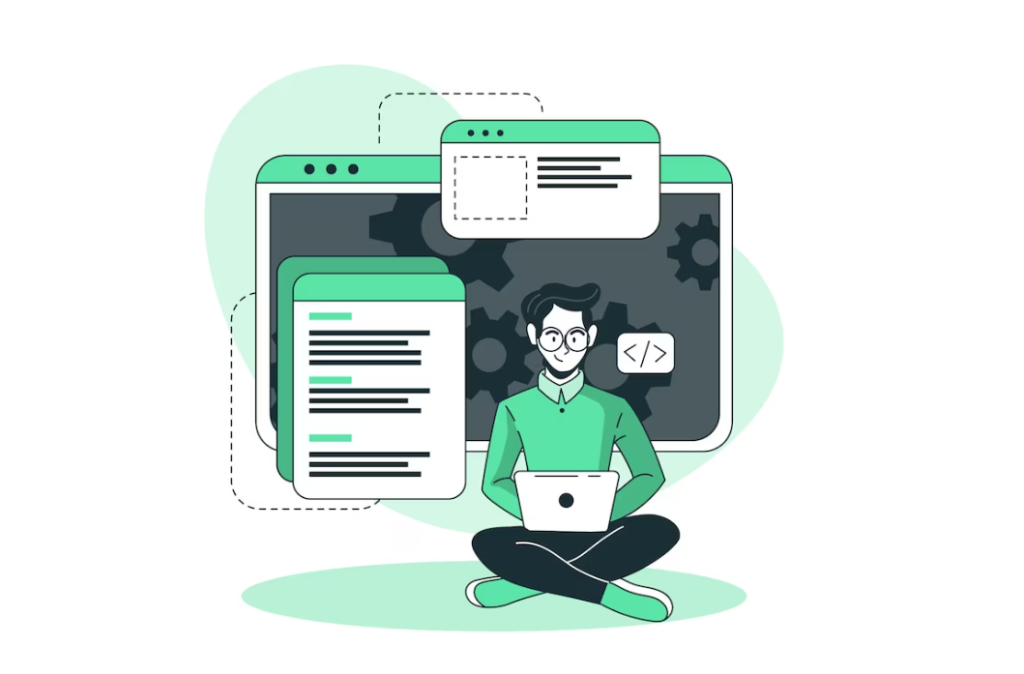
Button content Bottom Center position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: flex-end; /* vertical position */
align-items: center; /* horizontal position */
}
Button content Bottom Right position:
.button-container {
border: none;
border-radius: 0;
padding: 0;
background: deeppink;
color: white;
font-size: 16px;
width: 140px;
height: 45px;
display: inline-flex;
flex-direction: column;
justify-content: flex-end; /* vertical position */
align-items: flex-end; /* horizontal position */
}
Conclusion
Getting to grips with Flex Container CSS is a game-changer for any web developer or designer. This comprehensive toolset allows for a high degree of control over the positioning and layout of web content, permitting precise placement in nine main positions or even more. Whether working with nested elements or pure text content, Flexbox enables you to create responsive, visually appealing layouts that cater to diverse design needs.