Using CSS, you have the capability to enhance the visual aesthetics and user experience of HTML forms. This versatile styling language empowers you to create HTML forms that are not only visually appealing but also adaptable and refined in appearance. Throughout this tutorial, we will delve into a diverse array of CSS properties that can be harnessed for the purpose of crafting captivating and functional HTML forms.
Designing an Elegant and Responsive HTML Form with CSS
In this comprehensive guide, we’ll delve into designing a stylish and responsive HTML form using CSS. The form we aim to create will be visually appealing and functional across various device screens. Below, we’ll walk you through the step-by-step process, complete with code examples and valuable insights to ensure your form not only looks great but also functions flawlessly.
Understanding the Basic HTML Form Structure
Before we dive into the CSS styling, let’s take a closer look at the basic HTML form structure we’ll be working with. Understanding the components of your form is crucial before applying any styling. Here’s a breakdown:
<form action="" method="post">
<label for="name">Name</label><br>
<input type="text"><br>
<label for="email">Email</label><br>
<input type="email"><br>
<label for="age">Age</label><br>
<input type="number"><br>
<label for="country">Country</label><br>
<select id="country" name="country">
<option value="United States">United States</option>
<option value="India">India</option>
<option value="uae">United Arab Emirates</option>
<option value="Canada">Canada</option>
</select><br>
<label for="gender">Gender</label>
<input type="radio"> Male
<input type="radio"> Female
<br>
<label for="message">Message</label><br>
<textarea name="msg" id="message" cols="30" rows="10"></textarea><br>
<input type="submit">
</form>
Achieving Form Centering with CSS: Width and Margin
To ensure your web forms appear aesthetically pleasing and user-friendly, it’s crucial to define their width and position them at the center of the screen. In this section, we’ll explore how to do just that using CSS.
1. Setting Form Width:
The first step in this process is to determine the width of your form. By establishing an appropriate width, you can create a visually balanced form that fits neatly on the screen. Let’s get started:
<style>
form{
width: 50%;
margin: auto;
}
</style>
- Width Property: We set the width property to 50%, allowing the form to occupy half of the screen’s width. This creates a responsive design suitable for various device sizes;
- Margin Property: The margin property is set to ‘auto,’ which is a handy CSS trick for centering elements horizontally. It ensures that the form is equidistant from the left and right edges of the screen.
2. Centering the Form:
With the above CSS code, you’ve successfully centered your form on the screen. However, it’s worth noting that there are alternative methods for achieving this:
- Flexbox: Utilizing flexbox is another effective way to center elements. You can learn more about this technique and its advantages in our tutorial on “How to center a form in CSS.”;
- Grid Layout: CSS Grid offers powerful capabilities for layout control, including centering. Explore this option to gain more control over your form’s positioning.
Enhancing Your Form with Background Color and Padding
Now that your form is centrally positioned, it’s time to make it visually appealing by adding background color and padding. These style enhancements improve both aesthetics and user experience.
1. Adding Background Color:
A background color can create a clear distinction between your form and the surrounding content. Let’s apply a gray background color to your form:
<style>
form{
width: 50%;
margin: auto;
background-color: #f5f5f5;
}
</style>
Background-Color Property: Here, we set the background-color property to #f5f5f5, a shade of gray. Feel free to choose a color that matches your website’s design scheme.
2. Applying Padding:
Padding provides space between the content of your form and its borders, making it more visually appealing and user-friendly. Let’s add 50 pixels of padding to your <form> element:
Padding Property: The padding property is set to 50px, which creates a generous buffer around the form’s content. Adjust this value to achieve the desired spacing.
Expanding on CSS Form Font Size:
One of the key aspects of styling your HTML forms using CSS is controlling the font size. The font size greatly influences the readability and aesthetics of your form. By adjusting the font size property, you can make your form text look more appealing and user-friendly. Let’s delve deeper into this essential aspect of CSS form design.
Incorporating Font Size:
In the code snippet below, we’ll not only modify the font size but also provide additional tips on enhancing your form’s appearance. This CSS is applied to a form element, aiming to make it more visually appealing:
<style>
form {
width: 50%;
margin: auto;
background-color: #f5f5f5;
padding: 50px;
font-size: 20px;
}
</style>
Enhancing Styling for CSS Form Inputs:
Form inputs play a pivotal role in gathering user data, making them a critical element of any HTML form. Elevating the visual appeal and responsiveness of these inputs through CSS properties can significantly enhance the user experience. Let’s explore various CSS properties that can be applied to form inputs.
CSS Form Inputs Padding:
Padding, the inner spacing of an input field, not only affects the visual aesthetics but also impacts the overall usability of your form. In the following CSS code snippet, we apply padding to form inputs, making them more comfortable for users:
<style>
input, select, textarea {
padding: 10px;
}
</style>
CSS Form Inputs Width:
Another crucial aspect of form input styling is controlling their width. You can make form inputs appear wider, ideal for full-width input fields. The following CSS snippet demonstrates how to achieve this:
<style>
* {
box-sizing: border-box;
}
form {
width: 50%;
margin: auto;
background-color: #f5f5f5;
padding: 30px;
font-size: 20px;
}
input[type=text], input[type=email], input[type=number], select, textarea {
padding: 10px;
width: 100%;
}
input, select, textarea, label {
margin-bottom: 10px;
}
</style>
Managing Margin around CSS Form Inputs:
To fine-tune the spacing around your form input elements, the CSS margin property comes into play. In this section, we’ll explore how to set the bottom margin for every input field to create a visually pleasing and well-organized form.
<style>
input, select, textarea {
margin-bottom: 18px;
}
</style>
Enhancing Button Styling with CSS
When it comes to crafting visually appealing buttons on your website, CSS provides a versatile toolkit that lets you transform mundane buttons into eye-catching elements. By leveraging properties like padding, background color, and borders, you can create buttons that not only look great but also offer an improved user experience. Here’s how you can do it:
Padding: Adjusting the padding of a button can significantly impact its appearance and usability. Proper padding ensures that the button is not too cramped, making it easier for users to click on it. Additionally, it can create a sense of spaciousness and elegance. To apply padding to your button, follow these steps:
input[type=submit]{
padding: 12px 30px; /* top-bottom padding 12px, left-right padding 30px */
border: none;
background-color: #333333;
color: #ffffff;
font-size: 16px;
}
Background Color: Changing the background color of your button can instantly grab users’ attention and harmonize it with your website’s color scheme. The following CSS code snippet demonstrates how to alter the background color of a button:
input[type=submit]{
padding: 12px 30px;
border: none;
background-color: #333333; /* Change this color to your preferred shade */
color: #ffffff;
font-size: 16px;
}
Pro Tip: When selecting a background color, consider your brand’s color palette, user preferences, and readability. A well-chosen background color can make your button more inviting and clickable.
Customizing Form Input Borders
Enhancing the appearance of input fields in your forms is another essential aspect of web design. While the default input field borders are functional, you can make them more visually appealing by customizing them with CSS. Here’s how:
Use the border property to create a consistent border style for various input types, select elements, and text areas:
input[type=text], input[type=email], input[type=number], select, textarea{
border: 2px solid gray; /* Customize the border style to your liking */
}
Fine-tune individual sides of the border using border-right, border-left, border-top, and border-bottom properties for a unique look.
Add rounded corners to your input fields using the border-radius property:
input[type=text], input[type=email], input[type=number], select, textarea{
border: 2px solid gray;
border-radius: 5px; /* Adjust the radius value for your desired curvature */
}
Pro Tip: Creating rounded corners (border-radius) can soften the appearance of input fields and align them with modern design trends.
Elevating Input Fields with Background Color
The background color of your input fields can influence the overall aesthetics of your forms and contribute to a coherent design scheme. By carefully choosing background colors, you can make your forms more visually engaging. Here’s how to do it:
Set the background color for various input types, including text, email, number, select, and textarea:
input[type=text], input[type=email], input[type=number], select, textarea{
background-color: #D0F5BE; /* Select a color that complements your website's design */
}
Pro Tip: Consider using background colors that align with your brand identity and maintain good contrast with text for readability. A well-chosen background color can improve user interaction and overall user experience.
Enhancing User Interactivity with Focused Inputs
When it comes to creating engaging and interactive web forms, one essential feature you can leverage is “focused inputs.” Focused inputs add a touch of interactivity by triggering CSS styles when users click on input fields. Imagine the power of changing the background color or border of an input field as soon as it gains focus. This not only provides visual feedback to users but also enhances the overall user experience.
How to Implement Focused Inputs
To implement focused inputs in your web forms, you’ll need to utilize the :focus selector in your CSS code. Let’s delve into the specifics of creating this effect:
Consider a scenario where you want to change the background color of input fields from green to white when they are clicked. You can achieve this by applying the following CSS code:
input:focus{
background-color: #ffffff;
}
With this simple code snippet, you can transform the look and feel of your input fields, making your forms more engaging and user-friendly.
Expanding the Possibilities
While we’ve discussed changing the background color as an example, the same process applies to other CSS properties. You can alter various visual attributes, such as border color or text color, to further enhance user interactions.
Crafting Stylish and User-Friendly Forms
Creating a visually appealing and user-friendly form is crucial for any website. A well-designed form not only captures user data but also leaves a lasting impression. Let’s explore a comprehensive CSS code snippet that will take your form styling to the next level.
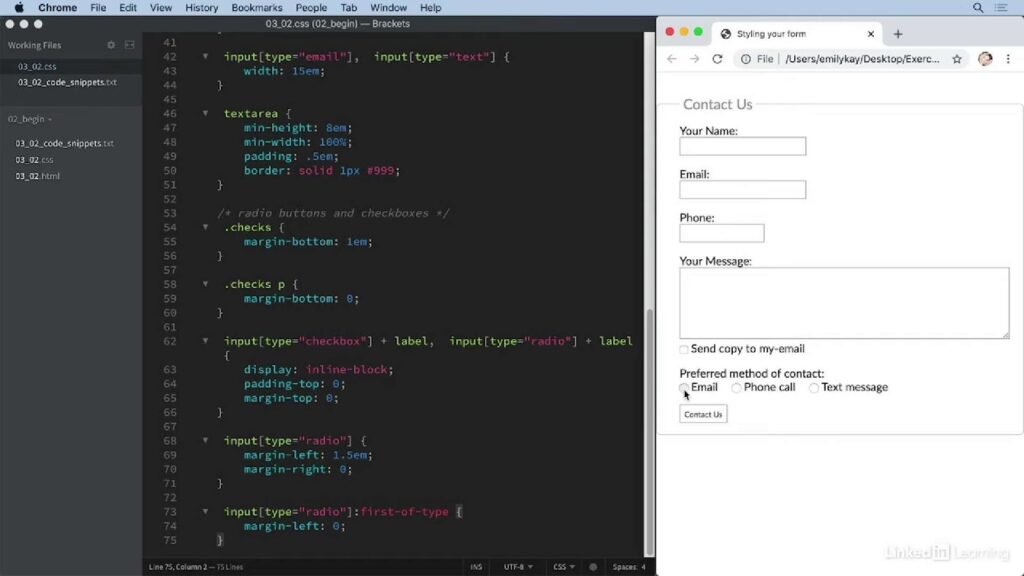
Optimizing Form Elements
Before diving into the CSS code, let’s optimize our form elements for a better user experience:
- Set a consistent font size (e.g., 16px) for all input fields and selects;
- Apply a box-sizing property with a value of border-box to ensure padding and borders are included in the element’s width and height calculation.
Form Styling CSS Code
Now, let’s take a closer look at the complete CSS code for styling your forms:
<style>
* {
box-sizing: border-box;
}
input, select {
font-size: 16px;
}
form {
width: 50%;
margin: auto;
background-color: #f5f5f5;
padding: 30px;
font-size: 20px;
}
input[type=text], input[type=email], input[type=number], select, textarea {
padding: 10px;
width: 100%;
border: 2px solid gray;
border-radius: 5px;
background-color: #D0F5BE;
}
input, select, textarea, label {
margin-bottom: 10px;
}
input[type=submit] {
padding: 12px 30px;
border: none;
background-color: #333333;
color: #ffffff;
font-size: 16px;
}
input, select, textarea {
margin-bottom: 18px;
}
</style>
Key Features of the CSS Code
Here are some key features and recommendations to consider when using this CSS code to style your forms:
- Box Sizing: The box-sizing property ensures that the padding and borders of form elements are included in their width and height calculation, preventing unexpected layout issues;
- Font Size: Setting a consistent font size (e.g., 16px) for input fields and selects ensures readability and a cohesive design;
- Background Styling: Customize the background color of input fields to match your website’s theme, enhancing visual appeal;
- Padding and Borders: Apply padding and borders to input fields, making them more visually distinct and user-friendly;
- Submit Button Styling: Make your submit button stand out with customized padding, background color, and text color.
By implementing this comprehensive CSS code, you’ll create forms that not only capture user data effectively but also leave a positive impression on your website visitors.
Conclusion
In conclusion, CSS offers a powerful toolset for elevating the design and functionality of HTML forms. By leveraging this versatile styling language, you can transform ordinary forms into visually appealing, responsive, and elegant elements of your web projects. Throughout this tutorial, we’ve explored a wide range of CSS properties that empower you to craft captivating and user-friendly HTML forms, ensuring a seamless and delightful experience for your website visitors.